Sword Weapon Code Implementation
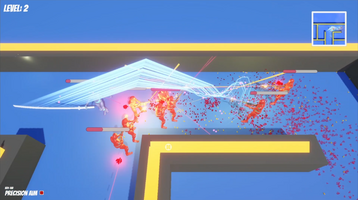
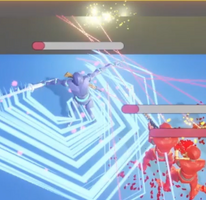
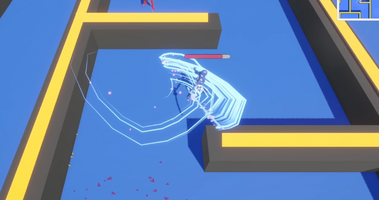
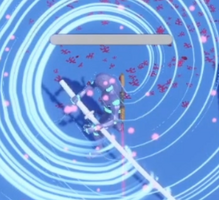
Overview
The Katana class implements the IWeapon
interface, providing functionality for equipping, using, and unequipping the melee weapon "Katana" in the game. This includes handling damage application and animation triggers for attacks.
Class Breakdown
Variables
-
weaponManager
: Reference to the weapon manager handling the current weapon. -
weaponData
: Data object storing information about the Katana (e.g., name, damage, etc.). -
thisMeleeWeaponHandler
: Handles melee weapon-specific actions, such as enabling colliders and detecting collisions.
Methods
1. EquipWeapon
-
Purpose: Prepares the Katana for use by replacing the current weapon, activating its collider, and subscribing to the collision detection event.
-
Parameters:
-
weaponManager
: Manages the equipped weapon. -
weaponUserType
: Indicates who is using the weapon (e.g., player or NPC). -
weaponSwitchType
: Defines how the weapon is switched (swapeHoldings
ordropPick
).
-
-
Key Actions:
-
Retrieves the Katana data from the inventory.
-
Replaces the current weapon with the Katana.
-
Activates the weapon collider for detecting collisions.
-
Subscribes the
GiveDamage
method to handle collision events.
-
2. Shoot
-
Purpose: Plays the attack animation when the Katana is used.
-
Actions:
-
Triggers the attack animation using
PlayWeaponAttackAnimation
from the weapon manager.
-
3. UnEquipWeapon
-
Purpose: Handles the unequipping of the Katana by disabling its collider and unsubscribing from collision events.
-
Parameters:
-
weaponUserType
: Indicates who is unequipping the weapon.
-
-
Key Actions:
-
Deactivates the weapon collider.
-
Unsubscribes the
GiveDamage
method from the collision event handler.
-
4. GetWeaponData
-
Purpose: Retrieves the Katana's weapon data.
-
Returns:
WeaponData
object containing weapon details.
5. GiveDamage
-
Purpose: Applies damage to objects that collide with the Katana during an attack.
-
Parameters:
-
other
: The game object that the Katana collides with.
-
-
Key Actions:
-
Checks the tag of the collided object to determine its type (player or enemy).
-
Calls appropriate damage functions for the player or enemy, passing the damage value and collision details.
-
Key Features
-
Dynamic Weapon Replacement: The
EquipWeapon
method ensures seamless replacement of the current weapon with the Katana. -
Collision-Based Damage Application: The
GiveDamage
method uses collision events to determine when and where to apply damage. -
Animation Integration: The
Shoot
method triggers the attack animation, enhancing player feedback and immersion.
Implementation Code
public class Katana : IWeapon { IWeaponManager weaponManager; WeaponData weaponData; MeleeWeaponHandler thisMeleeWeaponHandler; public void EquipWeapon(IWeaponManager weaponManager, WeaponUserType weaponUserType, WeaponSwitchType weaponSwitchType) { Debug.Log("EquipWeapon: Katana"); this.weaponManager = weaponManager; weaponData = WeaponInventory.instance.weaponsData.Find(ele => ele.weaponName == WeaponName.Katana); weaponManager.weaponHolder.ReplaceWeapon(weaponData, weaponSwitchType); thisMeleeWeaponHandler = weaponManager.weaponHolder.GetMeleeWeaponHandlerIfItsAMeleeWeapon(); // Setting the weapon collider enabled incase if its off thisMeleeWeaponHandler.SetTheColliderActive(true); // Subscribing the hit function thisMeleeWeaponHandler.OnAnyCollideEnter += GiveDamage; } public void Shoot() { Debug.Log("Shoot: Katana"); weaponManager.PlayWeaponAttackAnimation(weaponData); } public void UnEquipWeapon(WeaponUserType weaponUserType) { Debug.Log("UnEquipWeapon: Katana"); // Setting the weapon collider enabled false thisMeleeWeaponHandler.SetTheColliderActive(false); // Unsubscribing the hit function thisMeleeWeaponHandler.OnAnyCollideEnter -= GiveDamage; } public WeaponData GetWeaponData() { return weaponData; } public void GiveDamage(GameObject other) // This gives damage when weapon hit somewhere. { if (other.tag == Consts.playerTagString) { other.transform.parent.transform.GetComponent<PlayerController>().TakeDamage(weaponData.damage, other.transform.position); } else if (other.tag == Consts.enemyTagString) { // Give Damage to Enemy. other.transform.GetComponent<IEnemy>().TakeDamage(weaponData.damage, weaponManager.gameObject, other.transform.position); } } }
Notes
-
Ensure the
WeaponInventory
contains valid data for the Katana. -
Confirm that the
MeleeWeaponHandler
is correctly configured to handle colliders and collision events. -
Add appropriate functionality to
TakeDamage
methods in the player and enemy scripts for full integration.
Files
Maze Escape
Navigate the maze, grab the diamond, survive the hunt!
Status | Prototype |
Author | Goutamraj |
Genre | Action |
Tags | Singleplayer, Top down shooter |
More posts
- Diwali_Rifle Code Implementation😁38 days ago
- New Sword Weapon and Dash ability46 days ago
- Hit Effects and Mini Map53 days ago
- Player Movement Animations with Blend Tree59 days ago
- Add Precision Aim for Player and Update Guard Enemy with Laser Mechanics67 days ago
- Guard Enemy Implementation73 days ago
- Guard Enemy77 days ago
- Enemy Pool79 days ago
- Enemy Handling System81 days ago
Leave a comment
Log in with itch.io to leave a comment.