Player Movement Animations with Blend Tree
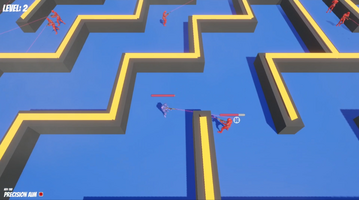
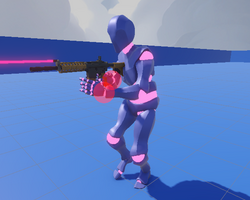
Player Movement Animations added. Let's discuss the implementation.
Player Animation Controller:
Overview
The Player Animation Controller script manages player animations based on input movements. It ensures smooth transitions and accurate alignment of the character's animations with the direction of movement.
Key Features
Dynamic Animation Updates: Animations adjust dynamically based on the player's movement input.
Local Space Movement Calculation: Translates 2D input into 3D space for precise animation control.
Animator Integration: Interacts seamlessly with Unity's Animator to manage animation parameters.
Script Breakdown
1. Variables
animator: A reference to the Animator component responsible for controlling animations.
2. Update Method
Calls CalculateAnimation each frame to update the animation parameters based on player input.
3. CalculateAnimation Method
Converts the 2D movement vector (Vector2) to a 3D vector (Vector3).
Computes local space movement using the dot product to determine the forward/backward and left/right movement.
Updates the animator's AxisZ and AxisX parameters to reflect movement direction and magnitude.
How It Works
- Input Handling: The script receives movement input as a 2D vector.
- 3D Conversion: Converts this input into a 3D vector suitable for animation calculation.
- Parameter Setting: Updates the Animator parameters, enabling animations to align with the player’s movement in local space.
Code Implementation
using UnityEngine; public class PlayerAnimationController : MonoBehaviour { [SerializeField] Animator animator; private void Update() { CalculateAnimation(PlayerInputsHandler.moveVector); } public void CalculateAnimation(Vector2 moveInput) { // Convert moveInput to a 3D vector (y = 0) Vector3 input3D = new Vector3(moveInput.x, 0, moveInput.y); // Calculate local space movement float forwardDot = Vector3.Dot(input3D, transform.forward); // Forward/backward float rightDot = Vector3.Dot(input3D, transform.right); // Right/left // Set animator parameters animator.SetFloat("AxisZ", forwardDot); // Y for forward/backward animator.SetFloat("AxisX", rightDot); // X for left/right } }
Animator Setup
The Animator Controller for the PlayerAnimationController uses a Blend Tree for smooth transitions between movement animations.
Structure:
-
Blend Tree Parameters:
-
AxisX
: Controls left-right movement. -
AxisZ
: Controls forward-backward movement.
-
Motion States:
The Blend Tree connects to five primary states:
-
Idle1: Player remains stationary.
-
RunForward1: Player moves forward.
-
RunBackward1: Player moves backward.
-
RunRight1: Player moves to the right.
-
RunLeft1: Player moves to the left.
Transition Logic:
-
Input Mapping:
-
AxisX
andAxisZ
values from theCalculateAnimation
method directly influence the Blend Tree to transition between states. -
Example: A positive
AxisZ
value transitions to the RunForward1 state.
-
Advantages of Blend Tree:
-
Smooth transitions between animations based on player input.
-
Minimal configuration for complex movement behaviors.
-
Precise control over animation speed and blending.
Maze Escape
Navigate the maze, grab the diamond, survive the hunt!
Status | Prototype |
Author | Goutamraj |
Genre | Action |
Tags | Singleplayer, Top down shooter |
More posts
- Diwali_Rifle Code Implementation😁40 days ago
- Sword Weapon Code Implementation46 days ago
- New Sword Weapon and Dash ability48 days ago
- Hit Effects and Mini Map55 days ago
- Add Precision Aim for Player and Update Guard Enemy with Laser Mechanics69 days ago
- Guard Enemy Implementation75 days ago
- Guard Enemy80 days ago
- Enemy Pool81 days ago
- Enemy Handling System83 days ago
Leave a comment
Log in with itch.io to leave a comment.