Guard Enemy Implementation
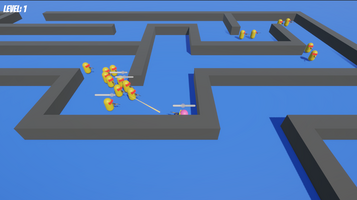
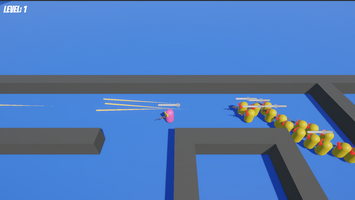
Killer Guard Enemy added.
Guard Enemy
The GuardEnemy
class is responsible for controlling the behavior of a guard-type enemy in the game. It handles the logic for patrolling, pursuing the player, taking damage, attacking, and managing the enemy's health and actions through coroutines and state transitions.
Class Overview
The GuardEnemy
class extends from IEnemy
and defines unique behaviors for a guard AI, including patrol movements, pursuit of the player, and attack mechanics. It interacts with various other systems, including weapon management and audio.
Serialized Fields
- navAgent (NavMeshAgent): Agent for pathfinding and navigation on the NavMesh.
-
petrolPointTransforms (List): List of patrol points for the enemy.
-
currentPatrolIndex (int): Index of the current patrol point.
- healthBar (Slider): UI element to display the enemy's health.
Script References
-
weaponManager_GuardEnemy (WeaponManager_GuardEnemy): Manages the guard's weaponry and firing actions.
-
enemyProvoker (Provoker): Handles the logic for when the enemy is provoked.
Values
-
isEngagged (bool): Indicates whether the enemy is engaged in pursuit or attacking.
-
isAttacking (bool): Indicates if the enemy is currently attacking the player.
-
patrollingSpeed (float): Speed at which the enemy patrols between points.
-
pursueSpeed (float): Speed at which the enemy chases the player.
-
maxAttackDistance (float): Maximum distance to engage the player in an attack.
-
minAttackDistance (float): Minimum distance to begin attacking.
UI
-
healthBarUI (Transform): The UI element's parent transform used for displaying the health bar.
Methods
Initialization
-
InjectManager(EnemyManager manager): Injects the manager responsible for controlling the enemy.
public override void InjectManager(EnemyManager manager) { base.InjectManager(manager); // Calls the base class's InjectManager method }
-
InitializeEnemy(EnemyManager enemyManager, Vector3 spawnPosition): Initializes the enemy's health, patrol points, and subscribes to provocation events.
public override void InitializeEnemy(EnemyManager enemyManager, Vector3 spawnPosition) { type = data.enemyType; health = data.health; healthBar.value = health / data.health; InjectManager(enemyManager); RegisterSelf(); petrolPointTransforms.Add(spawnPosition); // Additional patrol points are added here based on maze data commonEnemyStates = CommonEnemyStates.Patrolling; enemyProvoker.OnEnterProvokeRange += ProvokeMe; }
Health and Damage
-
TakeDamage(float damage): Decreases the health, shows the health bar, triggers provocation, and handles death.
public override void TakeDamage(float damage) { ProvokeMe(); // Starts pursuing the player when damage is taken health -= damage; healthBar.value = health / data.health; if (health <= 0) { // Handle enemy death UnregisterSelf(); EnemyPool.instance.ReturnEnemyObj(type, gameObject); } // Show health bar temporarily StartCoroutine(ShowHealthBarAndHideAfterSometime()); }
Provocation
-
ProvokeMe(): Starts the enemy’s pursuit of the player.
public override void ProvokeMe() { StartPursuing(); }
Patrolling
-
Patrol(): Moves the enemy to the next patrol point when the current point is reached.
public void Patrol() { if (petrolPointTransforms.Count == 0) return; if (!navAgent.pathPending && navAgent.remainingDistance < 0.5f) { CallGoToNextPetrollingPointAfterBeingIdealForSometime(); } }
-
CallGoToNextPetrollingPointAfterBeingIdealForSometime(): Initiates a delay before moving to the next patrol point.
public void CallGoToNextPetrollingPointAfterBeingIdealForSometime() { if (nextPetrolingPointIEnumerator != null) { StopCoroutine(nextPetrolingPointIEnumerator); } nextPetrolingPointIEnumerator = GoToNextPetrollingPointAfterBeingIdealForSometime(); StartCoroutine(nextPetrolingPointIEnumerator); }
Pursuing
-
StartPursuing(): Starts pursuing the player if the enemy is not already engaged.
public void StartPursuing() { if (isEngagged) return; isEngagged = true; navAgent.SetDestination(GameController.instance.playerController.transform.position); commonEnemyStates = CommonEnemyStates.Pursuing; StopPetrolling(); }
-
Pursue(): Handles the logic for the pursuit and attacks when the player is within range.
public void Pursue() { if (!isEngagged) return; float distance = Vector3.Distance(transform.position, GameController.instance.playerController.transform.position); if (distance > maxAttackDistance) { navAgent.SetDestination(GameController.instance.playerController.transform.position); Attack(false); // Stop attack if player moves out of range } else { if (distance < minAttackDistance) { navAgent.isStopped = true; Attack(true); // Start attack if within range } else { CheckBockingObstaclesWithRayCastAndPerformStopOrMove(); } } }
-
CheckBockingObstaclesWithRayCastAndPerformStopOrMove(): Uses raycasting to check if obstacles block the line of sight to the player.
public void CheckBockingObstaclesWithRayCastAndPerformStopOrMove() { RaycastHit hit; Vector3 directionToPlayer = (GameController.instance.playerController.transform.position - transform.position).normalized; bool hasLineOfSight = !Physics.Raycast(transform.position, directionToPlayer, out hit, maxAttackDistance, LayerMask.GetMask("Obstacle")); if (hasLineOfSight) { navAgent.isStopped = true; Attack(true); } else { navAgent.isStopped = false; navAgent.SetDestination(GameController.instance.playerController.transform.position); Attack(false); } }
Attacking
-
Attack(bool boolVal): Starts or stops the enemy's attack, depending on the provided boolean value.
public void Attack(bool boolVal) { isAttacking = boolVal; if (weaponManager_GuardEnemy.isfiring != boolVal) { weaponManager_GuardEnemy.isfiring = boolVal; if (boolVal) { weaponManager_GuardEnemy.Shoot(); } } }
-
RotatePlayerFaceTowardsPlayer(): Rotates the enemy to face the player while attacking.
private void RotatePlayerFaceTowardsPlayer() { if (isAttacking) { Vector3 aimDirection = (GameController.instance.playerController.transform.position - transform.position).normalized; Quaternion rotateTo = Quaternion.LookRotation(aimDirection, Vector3.up); transform.rotation = Quaternion.Slerp(transform.rotation, rotateTo, 0.15f); } }
UI
-
ShowHealthBarAndHideAfterSometime(): Coroutine to display the health bar briefly when damage is taken.
public IEnumerator ShowHealthBarAndHideAfterSometime() { healthBar.gameObject.SetActive(true); yield return new WaitForSeconds(10); healthBar.gameObject.SetActive(false); }
Reset
-
ResetMe(): Resets the enemy’s state, including patrol points, attack status, and UI.
public override void ResetMe() { petrolPointTransforms.Clear(); currentPatrolIndex = 0; isEngagged = false; isAttacking = false; weaponManager_GuardEnemy.isfiring = false; healthBarUI.gameObject.SetActive(false); }
Enemy States
The GuardEnemy
utilizes a state machine to manage different behaviors:
-
Idle: The enemy remains stationary.
-
Patrolling: The enemy moves between patrol points.
-
Alert: Placeholder for future behavior if required.
-
Pursuing: The enemy actively chases the player.
-
Investigating: Placeholder for future behavior if required.
Coroutines
-
GoToNextPatrollingPointAfterBeingIdealForSometime(): Waits for a random amount of time before moving to the next patrol point.
-
ShowHealthBarAndHideAfterSometime(): Displays the health bar for a brief period.
Key Behaviors
-
Patrolling: The enemy patrols between predefined points. Once a patrol point is reached, it moves to the next point after a random delay.
-
Pursuit: The enemy will pursue the player if provoked. The guard will attack if the player is within a defined range.
-
Attack: If the player is within the attack range, the enemy will stop moving and start attacking using its weapon manager. The enemy can rotate towards the player while attacking.
-
Health Management: The enemy has a health bar that decreases when taking damage. When health reaches zero, the enemy will die and be returned to the object pool.
Event Handling
-
OnEnterProvokeRange: When the player enters the provoke range, the enemy is triggered to engage and pursue the player.
Notes
-
The class makes use of
NavMeshAgent
for pathfinding, andWeaponManager_GuardEnemy
for weapon management. -
The class also handles the display of a health bar UI, which will appear when the enemy takes damage and disappear after a short time.
-
The
EnemyManager
is responsible for managing the enemy’s lifecycle and interactions with other game systems.
Summary
The GuardEnemy class provides a robust AI system with modular states for patrolling, pursuing, and attacking. It integrates seamlessly with external systems like pooling, navigation, and audio, ensuring reusability and efficient runtime behavior.
Check out this for code implementation: GuardEnemy
Files
Maze Escape
Navigate the maze, grab the diamond, survive the hunt!
Status | Prototype |
Author | Goutamraj |
Genre | Survival |
Tags | Singleplayer, Top down shooter |
More posts
- Add Precision Aim for Player and Update Guard Enemy with Laser Mechanics4 days ago
- Guard Enemy14 days ago
- Enemy Pool16 days ago
- Enemy Handling System18 days ago
- CellsPool (ObjectPool for Maze Cells)20 days ago
- Game Loop21 days ago
- Diamond Pickup22 days ago
- Entry and Exit path22 days ago
- Player State Machine25 days ago
Leave a comment
Log in with itch.io to leave a comment.